Excel表中每一行有规则 源目IP 源目端口 等等
有很多端口 客户那边说端不能重复创建 要单独服务 而且不能重复
例如 TCP53 UDP53 然后单独引用
我们拿出部分复制出来的端口
"22
80
2181-99999999
"53
123"
8018
53
"53
使用下方代码运行
import os
import re
def extract_and_combine_numbers(input_text):
# 提取数字
numbers = re.findall(r'\b\d+\b', input_text)
# 去重
unique_numbers = list(set(numbers))
# 将数字组成一个字符串,每个数字之间添加换行符
result_string = '\n'.join(unique_numbers)
return result_string
def process_file(input_file_path):
try:
with open(input_file_path, 'r') as file:
# 从文件中读取文本
input_text = file.read()
# 提取并组合数字
result = extract_and_combine_numbers(input_text)
# 构建输出文件路径
output_file_path = os.path.splitext(input_file_path)[0] + "_提取去重修改后.txt"
# 将结果写入新文件
with open(output_file_path, 'w') as output_file:
output_file.write(result)
return f"操作完成,结果保存在: {output_file_path}"
except Exception as e:
return f"发生错误: {str(e)}"
if __name__ == "__main__":
# 用户输入文件路径
print("请输入文件路径以文件名结束,例如 D:\\102\\123.txt")
file_path = input()
# 处理文件并输出结果
result_message = process_file(file_path)
print(result_message)
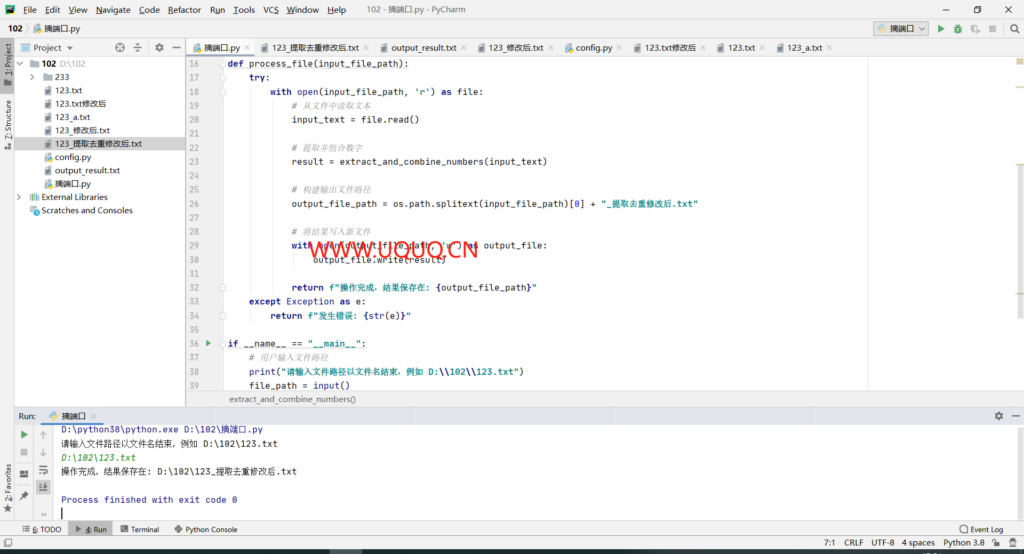
输出结果
99999999
53
22
80
123
2181
8018
缺点:大段 1-900 这种不能给他搞细 客户也说不用搞大段 小段直接手动搞 没几个
插入 将摘出来的端口 写插入进给他设置的脚本模板中
ip service-set XXX type object
service 0 protocol XXX source-port 0 to 65535 destination-port XXX
组合成功应该是下方这样的
ip service-set TCP2081 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 2081
ip service-set TCP2181 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 2181
ip service-set TCP2227 type object
写个小程序将他插入进去即可
import os
import re
def extract_and_generate_config(input_text, protocol):
# 提取每一行的数字
numbers = re.findall(r'\b\d+\b', input_text)
# 生成配置
config_lines = []
for number in numbers:
config_lines.append(f"ip service-set {protocol}{number} type object")
config_lines.append(f"service 0 protocol {protocol.lower()} source-port 0 to 65535 destination-port {number}")
return '\n'.join(config_lines)
def process_file(input_file_path, protocol):
try:
with open(input_file_path, 'r') as file:
input_text = file.read()
# 提取并生成配置
output_config = extract_and_generate_config(input_text, protocol)
# 构建输出文件路径,默认为输入文件所在目录
output_file_path = os.path.join(os.path.dirname(input_file_path), f"{os.path.splitext(os.path.basename(input_file_path))[0]}_{protocol.lower()}_插入后.txt")
# 将生成的配置写入新文件
with open(output_file_path, 'w') as output_file:
output_file.write(output_config)
return f"操作完成,插入的文件保存在: {output_file_path}"
except Exception as e:
return f"发生错误: {str(e)}"
if __name__ == "__main__":
# 用户输入文件路径
print("请输入文件路径以文件名结束,例如 D:\\102\\123.txt\n")
file_path = input()
# 用户选择TCP或UDP
print("请选择协议:")
print("1. TCP")
print("2. UDP")
protocol_choice = input("请输入选项 (1 或 2): ")
if protocol_choice == '1':
protocol = "TCP"
elif protocol_choice == '2':
protocol = "UDP"
else:
print("无效的选项,程序退出。")
exit()
# 处理文件并输出生成的配置,默认输出到输入文件所在目录
result_message = process_file(file_path, protocol)
print(result_message)
输出
ip service-set TCP99999999 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 99999999
ip service-set TCP53 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 53
ip service-set TCP22 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 22
ip service-set TCP80 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 80
ip service-set TCP123 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 123
ip service-set TCP2181 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 2181
ip service-set TCP8018 type object
service 0 protocol tcp source-port 0 to 65535 destination-port 8018
下一步 我们在写入规则时 调用这些
比如说rule name xxx
rule name XXX
service TCP99999999
service TCP53
service TCP22
咱们在前面添加进去 service TCP刷入即可
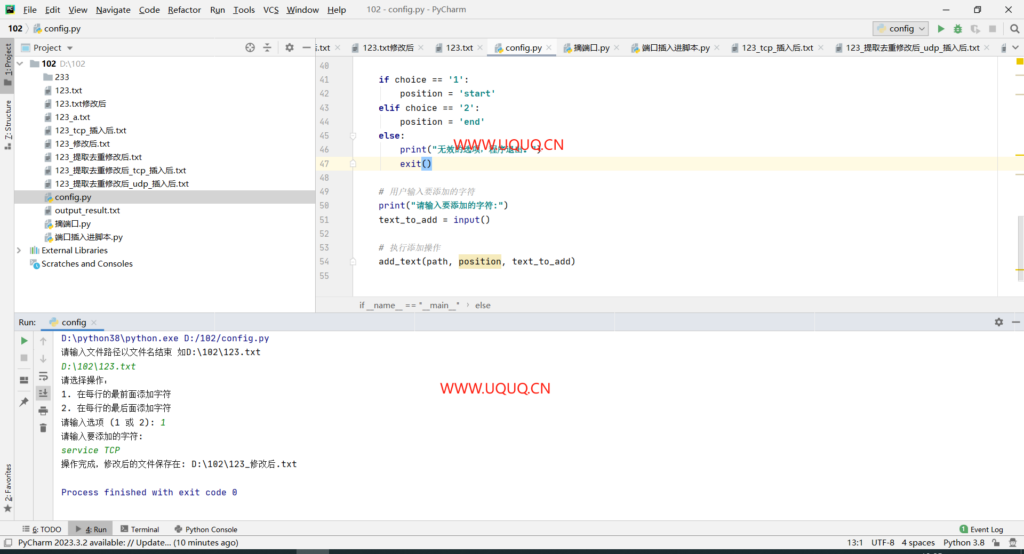
import os
def add_text(file_path, position, text_to_add):
# 提取文件名
file_name = os.path.basename(file_path)
# 提取路径
path_a = directory_path = os.path.dirname(file_path)
# 打开文件并读取内容
with open(file_path, 'r') as file:
lines = file.readlines()
# 在每一行的指定位置添加文本
if position == 'start':
modified_lines = [text_to_add + line if line.strip() else text_to_add + line for line in lines]
elif position == 'end':
modified_lines = [line.rstrip() + text_to_add + '\n' for line in lines]
# 生成添加后的文件
modified_file_name = f"{os.path.splitext(file_name)[0]}_修改后.txt"
modified_file_path = os.path.join(path_a, modified_file_name)
with open(modified_file_path, 'w') as file:
for line in modified_lines:
file.write(line)
print("操作完成,修改后的文件保存在:", modified_file_path)
if __name__ == "__main__":
# 用户输入文件路径
print(r"请输入文件路径以文件名结束 如D:\102\123.txt")
path = input()
# 在最前面或最后面添加的字符
print("请选择操作:")
print("1. 在每行的最前面添加字符")
print("2. 在每行的最后面添加字符")
choice = input("请输入选项 (1 或 2): ")
if choice == '1':
position = 'start'
elif choice == '2':
position = 'end'
else:
print("无效的选项,程序退出。")
exit()
# 用户输入要添加的字符
print("请输入要添加的字符:")
text_to_add = input()
# 执行添加操作
add_text(path, position, text_to_add)
输出
service TCP99999999
service TCP53
service TCP22
service TCP80
service TCP123
service TCP2181
service TCP8018
端口部分这样搞就节省很多时间了
由于此次配置文档里面 部分IP地址是写的中文名称 没直接写IP地址,以及还有部分用不到的 ,所以地址组没用Python偷懒。。。。下次遇到在整吧
评论 (0)